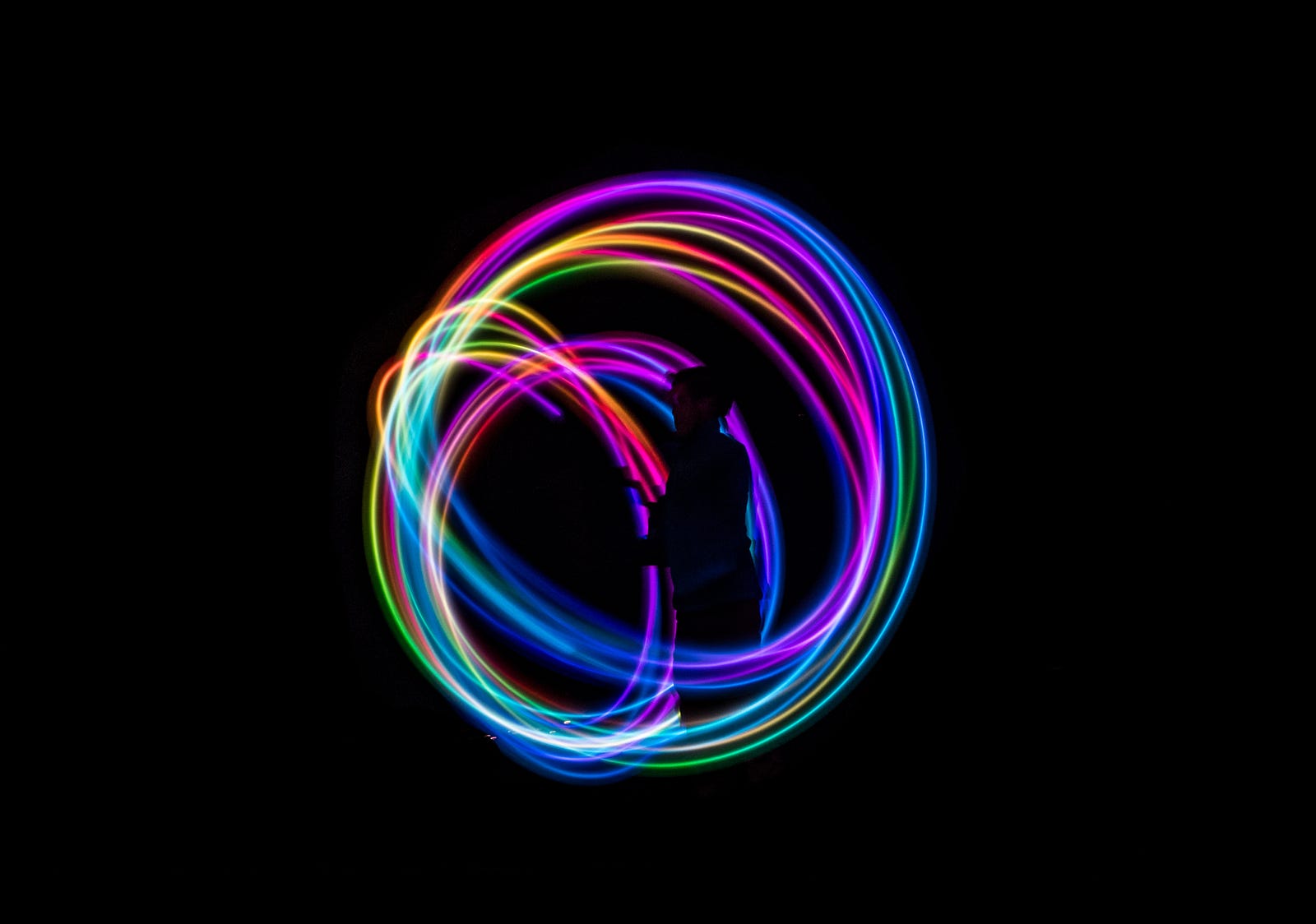
Introduction
You’re probably here because your app has grown to the point where forcing the user to download the entire thing as a single file seems cruel and unusual. Fact is, tons of features and a complex UX are bound to affect the amount of code you’re working with. What to do?
Easy. Make a “vendor bundle.” A vendor bundle contains all the frameworks and libraries each application feature depends on. By building all this code into a single bundle, the client can effectively cache the bundle, and you only need to rebuild the bundle when a framework or library updates.
It’s also worth considering that every time your application is updated or changed, the client must download new vendor dependencies. What to do?
You guessed it. Bundle ’em up. Put the parts that changed in one bundle and the dependencies in another. That way, the client only downloads what it needs.
In this post, I’ll walk you through the basics of bundle splitting in Webpack. As you might expect, I’ll also touch on code splitting.
How to create a vendor bundle
Assuming you have a basic React application, execute npm run build
in CLI to get a baseline build:
Hash: 9db5a05e09ad73897fd4 Version: webpack 2.2.1 Time: 2114ms Asset Size Chunks Chunk Names ...font.eot 166 kB [emitted] ...font.woff2 77.2 kB [emitted] ...font.woff 98 kB [emitted] ...font.svg 444 kB [emitted] [big] logo.png 77 kB [emitted] ...font.ttf 166 kB [emitted] app.js 170 kB 0 [emitted] app app.css 3.11 kB 0 [emitted] app app.js.map 193 kB 0 [emitted] app app.css.map 89 bytes 0 [emitted] app index.html 248 bytes [emitted] [0] ./~/process/browser.js 7.3 kB {0} [built] [3] ./~/react/lib/ReactElement.js 13.2 kB {0} [built] [18] ./app/component.js 292 bytes {0} [built] ...
As you can see, theapp is quite large — about 170kb. That’s something you’ll fix in our upcoming example.
Code splitting
Code Splitting is an awesome feature that splits your codebase into various, smaller bundles which can be loaded whenever they are needed.
What this means is that your application is composed of lots of small code files (as modules) which are called “chunks”. If used properly, code splitting will lower loading time. Webpack allows you to define split points in your code base and then takes care of the dependencies, output files and runtime stuff.
Let’s proceed to walk through the most common approaches to code splitting when using Webpack.
Asynchronous Loading
This is just basically splitting code by loading the modules asynchronously. Say you have this directory with Lodash in the node_modules
:
webpack-demo
|- package.json
|- webpack.config.js
|- /dist
|- /src
|- index.js
|- /node_modules
In the index.js
file, import lodash
using Common JS require.ensure
. As the method name goes, it ensures that the module is only loaded when the code is required and it does this asynchronously:
require.ensure(['lodash'], function() { var lodash = require('lodash'); ... })
You don’t need to change anything in the Webpack configuration:
const path = require('path'); const HTMLWebpackPlugin = require('html-webpack-plugin'); module.exports = { entry: { index: './src/index.js' }, plugins: [ // ... ], output: { filename: '[name].bundle.js', path: path.resolve(__dirname, 'dist') } };
Running npm run build
in our CLI will yield the following build result:
Hash: a27e5bf1dd73c675d5c9 Version: webpack 2.6.1 Time: 544ms Asset Size Chunks Chunk Names lodash.bundle.js 541 kB 0 [emitted] [big] lodash index.bundle.js 6.35 kB 1 [emitted] index [0] ./~/lodash/lodash.js 540 kB {0} [built] [1] ./src/index.js 377 bytes {1} [built] [2] (webpack)/buildin/global.js 509 bytes {0} [built] [3] (webpack)/buildin/module.js 517 bytes {0} [built]