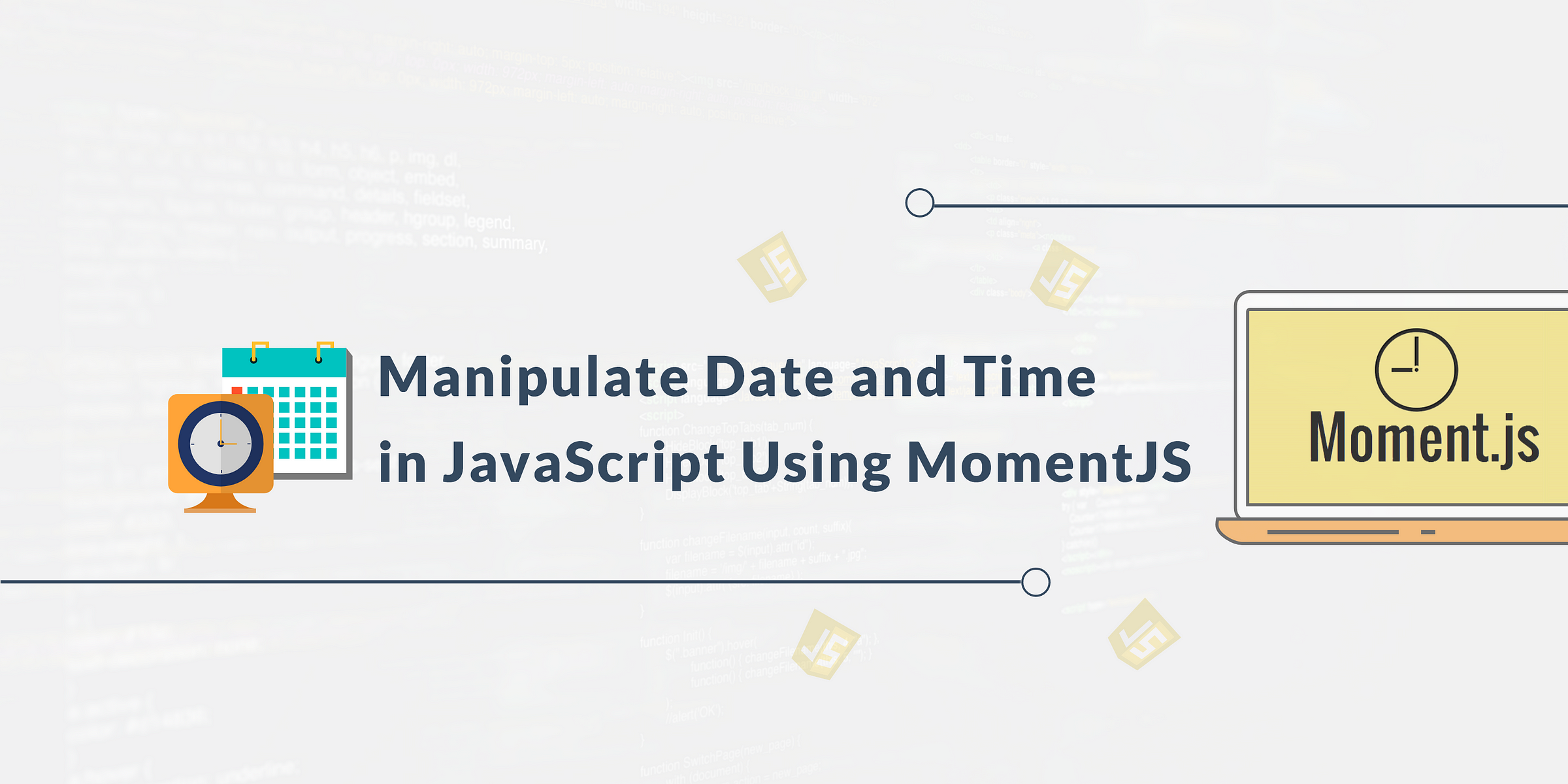
It’s almost 2018! We use dates and time every day of our lives, I just did, and if you are a developer designing a product, tool, or service, you most likely would need to manipulate date in whatever you are building.
How do you work with dates in your script? new Date()
? Manipulating like that can be, at best, cumbersome. Moment is a JavaScript library that, with its approachable API methods, makes working with dates a lot easier.
In this tutorial, I‘ll discuss working with moment
to effectively manipulate date and time in JavaScript.
Installation
Moment
can be run on both the browser as well as on the server-side. Using Node.js on the server-side, moment is installed via npm by running this on the command line:
npm install -g moment
moment
can then simply be required:
var moment = require('moment') var today = moment().format()
We just created the moment
object, which can be manipulated to return any desired date value. Once the moment function is called with moment()
it defaults to the current date, just like new Date()
.
In the browser, moment
is dowloaded here and included in a script tag like this:
<script src="moment.js"></script>
Alternatively, it can be imported from a CDN and used as a source in script tags. Other installation methods can be found here.
Manipulating date and time
The moment
object gives us the ability to manipulate the date object however we choose, applying several (chaining) moment
methods to it returns desired formatted date values.
For example, we get the following format with new Date()
:
new Date(); // Wed Oct 18 2017 19:36:39 GMT+0100 (WAT)
What if in our use-case, it’s more intuitive to show the user a relative date? With moment, you can simply do this:
var then = moment('2010-09-17').fromNow() // "7 years Ago"
Calling moment()
without any parameters returns the current date in your timezone.
var today = moment() // returns today's date in current timezone
Let’s go in-depth with more formatting approaches that Moment offers.
Date formatting
Previously, formatting dates with the Date()
string involved performing several string operations on the returned date string. With moment
we just need to add the format()
method to format the date. This method also receives parameters defining how we would like the date to appear.
var today = moment().format() //returns 2017-10-04T21:07:52+01:00 var today = moment().format('YYYY-MM-DD') //returns 2017-10-04 var today = moment().format('YY/MMM/DD') // returns 17/Oct/04 var today = moment().format('D/MMMM/Y') // returns 4/October/17 var time = moment().format('ddd DD/MM/YYYY') //returns Wed 04/10/2017
To get a particular moment
as a string, pass the string value as a parameter when calling moment()
.
var time = moment('2017/10/5') // returns Thu Oct 05 2017 00:00:00 GMT+0100
The format of the date specified can also be passed in as a parameter if known. The date is treated as invalid
if its values cannot be parsed.
var time = moment('2017/10/5', 'YYYY/DD/MM')// returns Wed May 10 2017 00:00:00 GMT+0100
Others date formats can be found here.
moment
uses method chaining to do awesome manipulations when a moment
is called, for instance:
var newMoment = moment().add().subtract().fromDate()
This is just an illustration of chaining methods in moment
. Also note that moments are mutable and therefore change the original fetched moment
. To create a new manipulated moment
, the original moment
can be cloned into a new variable.
Subtract
This method subtracts time from the original moment. Here, the subtract()
method is called and passed parameters of a number denoting the amount of time to be removed and the time value. This time value can also be written in shorthand, they include:
- d: Days
- y: Years
- Q: Quarters
- M: Months
- w: Weeks
- h: Hours
- m: Minutes
- s: Seconds
- Ms: Milliseconds
moment().subtract(val, string)
var lastWeek = moment().subtract(7, 'days') //subtracts 7 days from the current date
Multiple combinations can also be done with the subtract time method by chaining.
var someWeek = moment().subtract(1, 'week').subtract(2, 'days').subtract(5, 'weeks')
Add
This is similar to the subtract()
method and only adds a desired amount of time to the moment
.
moment().add(val, string)
var nextHour = moment().add(1, 'hour')
Start of
This method mutates the moment
to the start of a specified time. This sets the time to the beginning of the current time, either to the beginning of the day, month, year, or minute.
var time = moment().startOf('day') // returns time at beginning of the day - 12:00am var time = moment().startOf('week')// Uses the locale aware start of week day var time = moment().startOf('year') var time = moment().startOf('minute')
End of
This is the opposite of startOf()
and mutates the moment
to the end of a unit of time. This includes the week, month, day, or year.
var endOfMonth = moment().endOf('Month') // Returns the last time at the end of the month.
Time from now
This displays relative time is used to give a whole picture of the difference between two timeframes.
var then = moment('2010-09-17').fromNow() //returns "7 years Ago" var justNow = moment().fromNow() //returns "A few seconds ago"
The fromNow()
method also takes a boolean, passing in true
removes the ‘ago’ suffix.
var since = moment("27/08/2017", "DD/MM/YYYY").fromNow(true) // a month
To view the exact time from a particular date, the from()
method is used.
More great articles from LogRocket:
- Don't miss a moment with The Replay, a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
var time = moment("27/08/2017", "DD/MM/YYYY").from(moment('27/09/2017', "DD/MM/YYYY")) //a month ago
Time to now
Similar to fromNow()
, the toNow()
method returns the opposite of the fromNow()
method. The to()
method is also used to show relative time between two time values.
var time = moment("27/08/2016", "DD/MM/YYYY") var time2 = moment("27/09/2017", "DD/MM/YYYY") console.log(time.to(time2)) // in a year var time2 = moment("27/09/2017", "DD/MM/YYYY") console.log(time2.toNow())// in 8 days
Difference
This is one of the common problems encountered when manipulating time and date. With moment
, the diff()
method is used to calculate the difference between two moments and it returns the difference in milliseconds. However, other units of time such as years, months, weeks, days, hours, minutes, and seconds can be returned. The diff()
method takes in a unit of time as a first parameter and can take the desired time unit as a second parameter.
momentA.diff(momentB, 'unit')
var time = moment("27/08/2018", "DD/MM/YYYY") var time2 = moment("27/09/2017", "DD/MM/YYYY") console.log(time.diff(time2,"days") + ' days') // 334days
Query
These are moment
methods that return boolean values and is used to compare two date values.
isBefore()
As its name implies, the isBefore()
method verifies if one date value is before another date value.
var then = moment("27/08/2018", "DD/MM/YYYY") var now = moment("27/09/2017", "DD/MM/YYYY") console.log(then.isBefore(now)) // false console.log(now.isBefore(then)) // true
isAfter()
Similar to the isBefore()
method, this method verifies if a date after is after another date value and returns either true
or false
.
var time = moment("27/08/2019", "DD/MM/YYYY") var time2 = moment("27/12/2018", "DD/MM/YYYY") document.write(time.isAfter(time2)) // true
Other query methods used in comparing two date values include:
- isSame()
- isBetween()
- isSameOrAfter()
- isSameOrBefore()
These methods all follow the same syntax and return true
or false
. There are also methods which verify the validity of a certain date. These are:
isDaylightSavingTime
The isDST()
verifies if the moment
is in Daylight Saving Time or not.
var time2 = moment("27/09/2017", "DD/MM/YYYY").isDST() console.log(time2) // false
isLeapYear
The isLeapYear()
method verifies if a year is a leap year and returns a boolean.
var time = moment(2012, "YYYY").isLeapYear(); console.log(time) //returns true
isValid
With the isValid()
method, the validity of a moment
can be ascertained.
var time = moment('2012/34/28', "YYYY/MM/DD").isValid(); //returns false console.log(time) //returns false var time = moment('2012/02/18', "YYYY/MM/DD").isValid(); console.log(time) //returns true
Conclusion
In this article, we have seen how moment
make date and time handling and management seamless. By simply applying methods on the moment
object either singularly or in chains we can effectively manipulate our date and time to achieve desired results.
Find additional resources here on the official moment.js documentation.